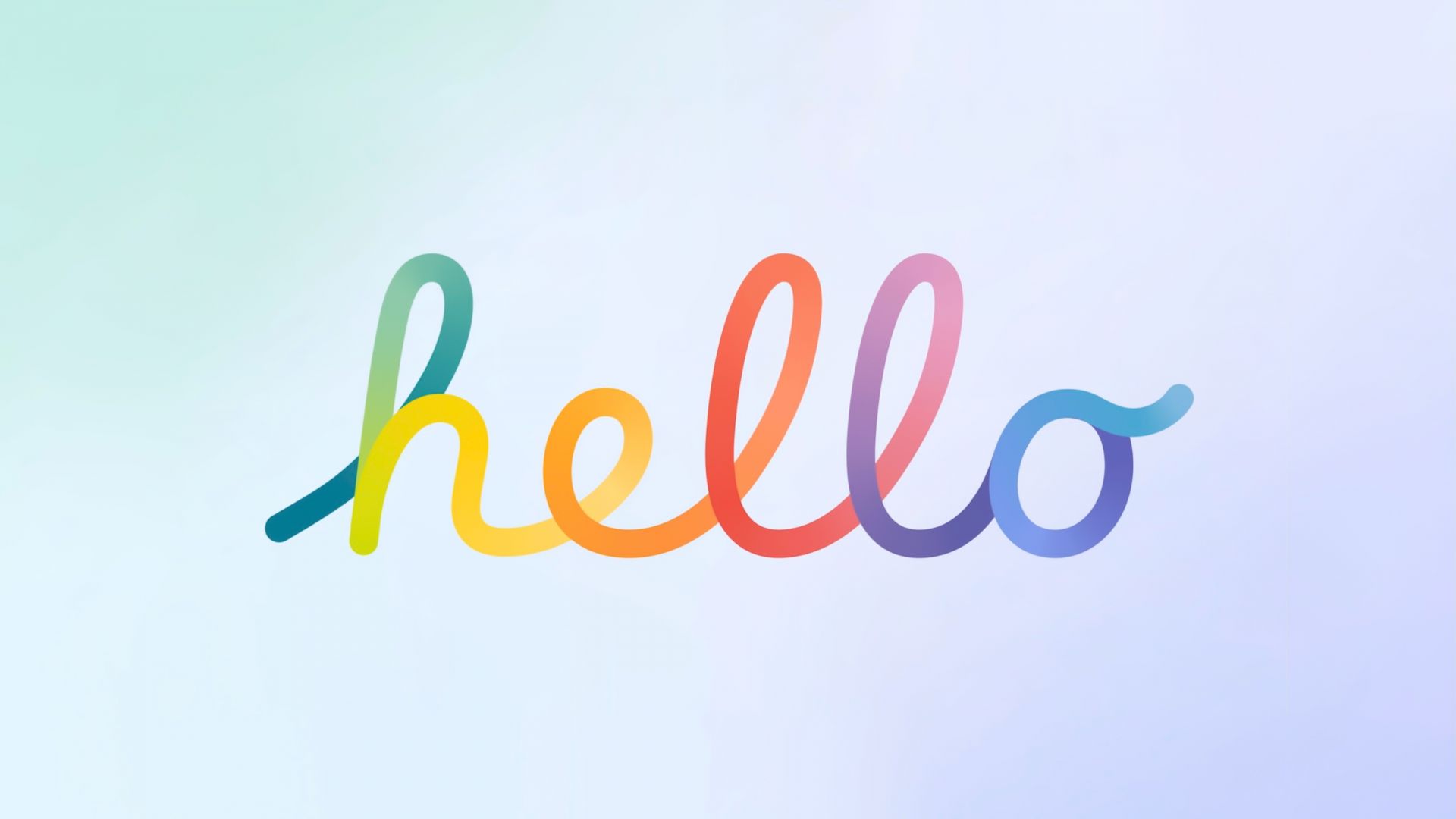
小程序全局数据共享
小程序全局数据共享
为了解决组件之间数据共享的问题
安装包
小程序全局数据共享需要使用到两个 npm 包
1 | npm i mobx-miniprogram mobx-miniprogram-bindings -S |
- mobx-miniprogarm:用来创建 Store 对象
- mobx-miniprogarm-bindings:把 Store 对象中的数据和方法绑定到组件或页面上
创建实例对象
在项目根目录下创建 store 文件夹,全部的 mobx 相关的文件都需要放在这个文件夹中
在 store 文件夹中创建 store.js
1 | import { |
创建数据和方法
数据
1 | import { |
计算属性
1 | import { |
方法
1 | import { |
绑定数据和方法
在页面组件中
1 | import { |
createStoreBindings:创建实例对象
- 第一个参数(this):指代当前组件实例对象
- 第二个人参数:配置项
- store:数据源
- fields:需要导入的数据或计算属性
- actions:需要导入的方法
destroyStoreBindings:卸载实例对象
在自定义组件中
1 | import { |
-
感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 View Room
评论